How I monitor and maintain over 40 third party integrations at work
My Job
As a Senior Integrations Engineer at involve.me, I lead the development and maintenance of over 40 third-party integrations that enable our AI-powered platform to help businesses turn website traffic into qualified leads. Our platform thrives on personalization and interactivity at every stage of the customer journey.
Why do I need to monitor all these integrations?
Managing such a large volume of integrations presents unique challenges. Despite having more than 150 automated integration tests and over 500 assertions, there are still edge cases where it's hard to replicate issues reported by specific users, trace slowdowns in certain integrations, or pinpoint exactly where failures occur.
To solve these problems, I need to instrument every integration request.
Instrumenting requests means adding code or tools to track, log, monitor, or analyze the requests your application sends or receives for visibility, performance, debugging, and observability.
You’re basically telling your application: “Hey, every time a request happens, let me know what, when, how long, and whether it succeeded or failed.”
What follows is a deep technical dive into how we instrument every request and gain full observability across our integration layer, but first, I must initially explain some terms for you to understand my approach to solving this problem.
HTTP Requests
An HTTP request is a message that a client (like your browser or an app) sends to a server asking for something, usually a web page, data, or to perform some action.
HTTP stands for HyperText Transfer Protocol, and it's the foundation of how data is exchanged on the web.
You interact with an API by sending HTTP requests to it.
Laravel
Laravel is a popular PHP web framework used to build modern web applications. It is a toolkit that helps developers write clean, structured, and efficient code, especially for backend systems like APIs, web apps, or complex business logic.
Read more about this here.
Sentry
Sentry is a real-time error-tracking and performance monitoring tool for developers. It helps you identify, fix, and monitor bugs and issues in your applications across frontend, backend, and mobile platforms.
Read more about this here.
Saloon
Saloon is a PHP library that helps you rapidly build third-party API integrations or SDKs. It is production-ready by default and offers a simple, standardised development flow.
Read more about this here.
Laravel, Saloon, and HTTP Requests
To install Saloon in your Laravel application, run the following command:
composer require saloonphp/saloon "^3.0"
Read more about this here.
To install the Laravel plugin:
composer require saloonphp/laravel-plugin "^3.0"
Read more about this here.
The plugin includes facades and console commands to help you get started quickly.
Creating a Saloon Connector and Request
A typical Saloon HTTP request involves creating a connector and a request class. The connector defines the base URL and common configurations. The request defines the endpoint, method, and payload.
Connector Example
<?php
use Saloon\Http\Connector;
class ExampleConnector extends Connector
{
public function resolveBaseUrl(): string
{
return 'https://example.com/api/v1';
}
}
Read more about this here.
Request Example
<?php
use Saloon\Enums\Method;
use Saloon\Http\Request;
class GetExampleListsRequest extends Request
{
protected Method $method = Method::GET;
public function resolveEndpoint(): string
{
return '/lists';
}
}
Read more about this here.
Sending a Request
<?php
$example = new ExampleConnector;
$request = new GetExampleListsRequest;
$response = $example->send($request);
Read more about this here.
Adding Sentry Middleware to Saloon
Saloon provides a middleware system that allows you to tap into the request and response lifecycle, enabling you to modify the request before it is sent or process the response after it is received.
You need to set up Sentry in your Laravel app first. To do this, follow the documentation here.
Instrumenting HTTP Requests
To instrument your HTTP requests with Sentry, update your connector constructor:
$this->sender()->addMiddleware(GuzzleTracingMiddleware::trace(), 'any-label-you-like');
This adds the Sentry middleware to every outgoing Saloon HTTP request.
Read more about the Sentry middleware here.
Read more about Saloon Guzzle Instance here.
Result
Once configured, your Sentry dashboard will display:
HTTP method and URL
Request latency
Success or error status
Exception traces
Overall throughput and performance metrics
This setup provides end-to-end observability for your Laravel application's HTTP requests.
See the screenshot below for a typical example.
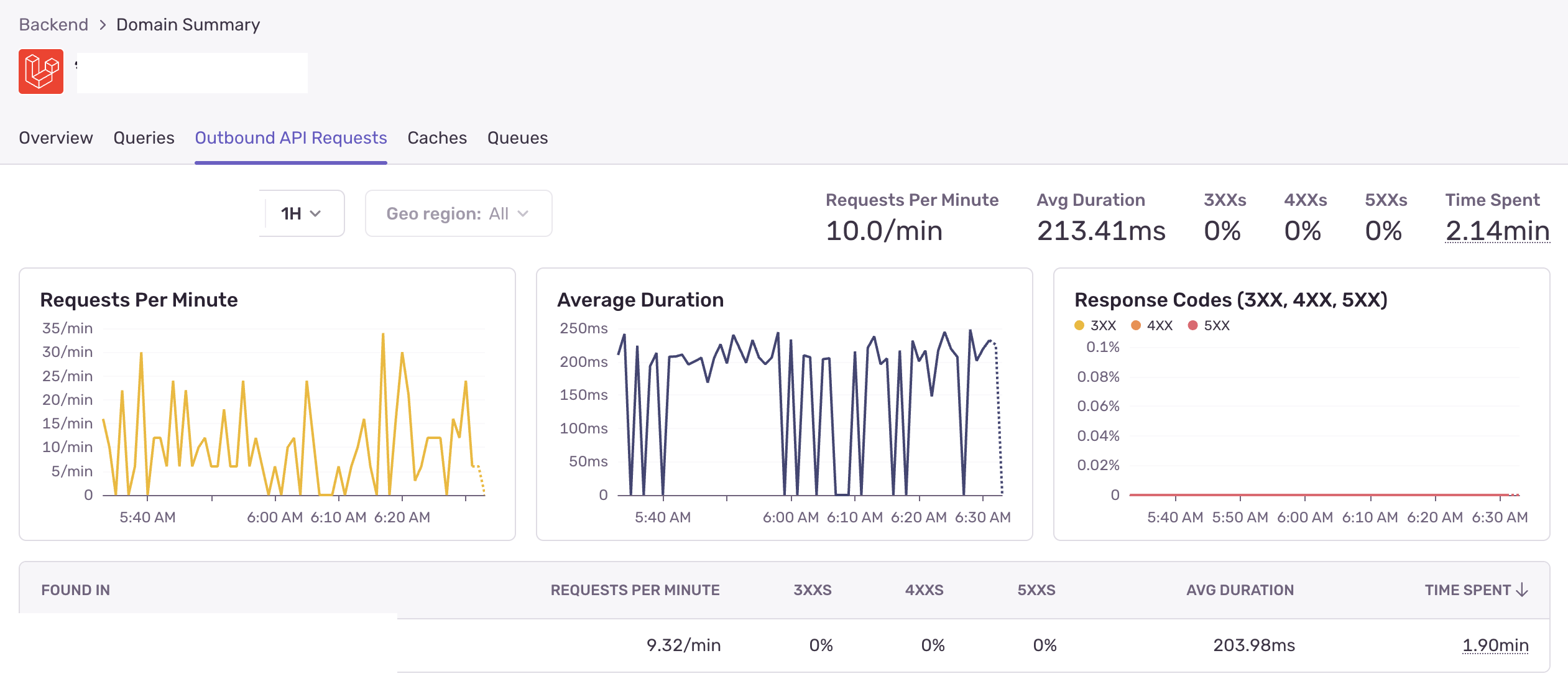
Takeaways
Keeping track of a bunch of integrations can get overwhelming, but with the right monitoring system (not just Sentry), it's a breeze!